Sample Code for Hardware Components on QNX Pi
Start your hardware project quickly with these samples showing how to use various hardware components with QNX 8.0 on a Raspberry Pi.
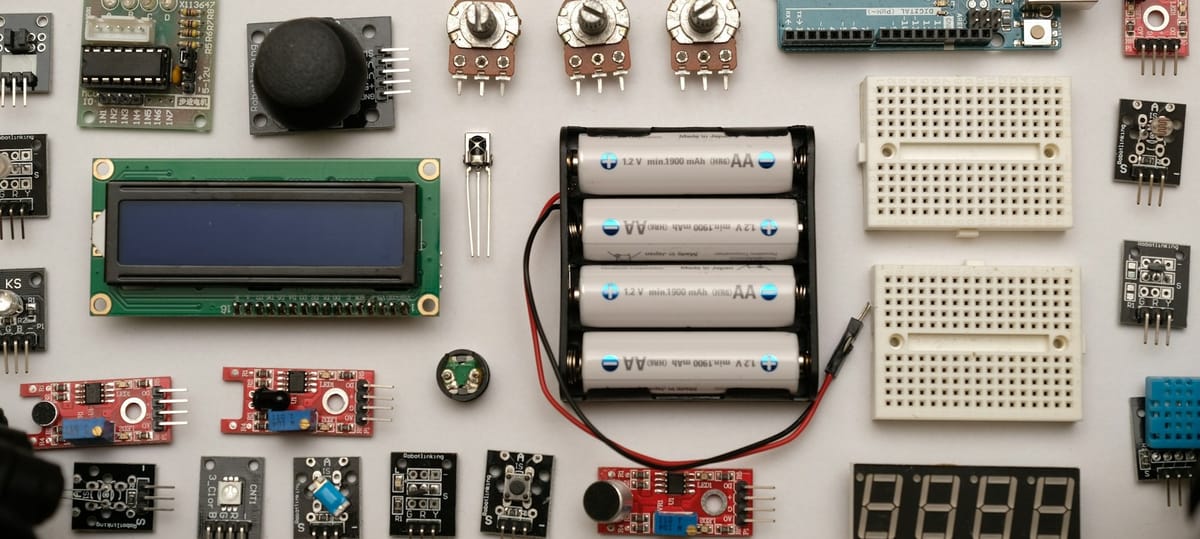
At QNX we're just getting started with writing and publishing sample code to help you accomplish all types of goals with QNX. Today I'd like to share some of the recent sample code we've made available: basic samples to show how to use some different hardware components with Raspberry Pi running QNX.
First-up: The Trilobot
The Trilobot from Pimoroni is a versatile, mid-level robot learning platform designed for use with Raspberry Pi. It's built with educational purposes in mind, making it straightforward to get started while allowing for easy expansion and customization. It features Qwiic connectors and breakout pads for expanding the platform with whatever you need.
On the platform you'll find:
- An ultrasonic sensor
- Underbelly RGB LEDs
- Two motors with grippy wheels
- Four buttons to help provide input
- Space for a Pi camera
- Qwiic connectors and breakouts to add your own gear
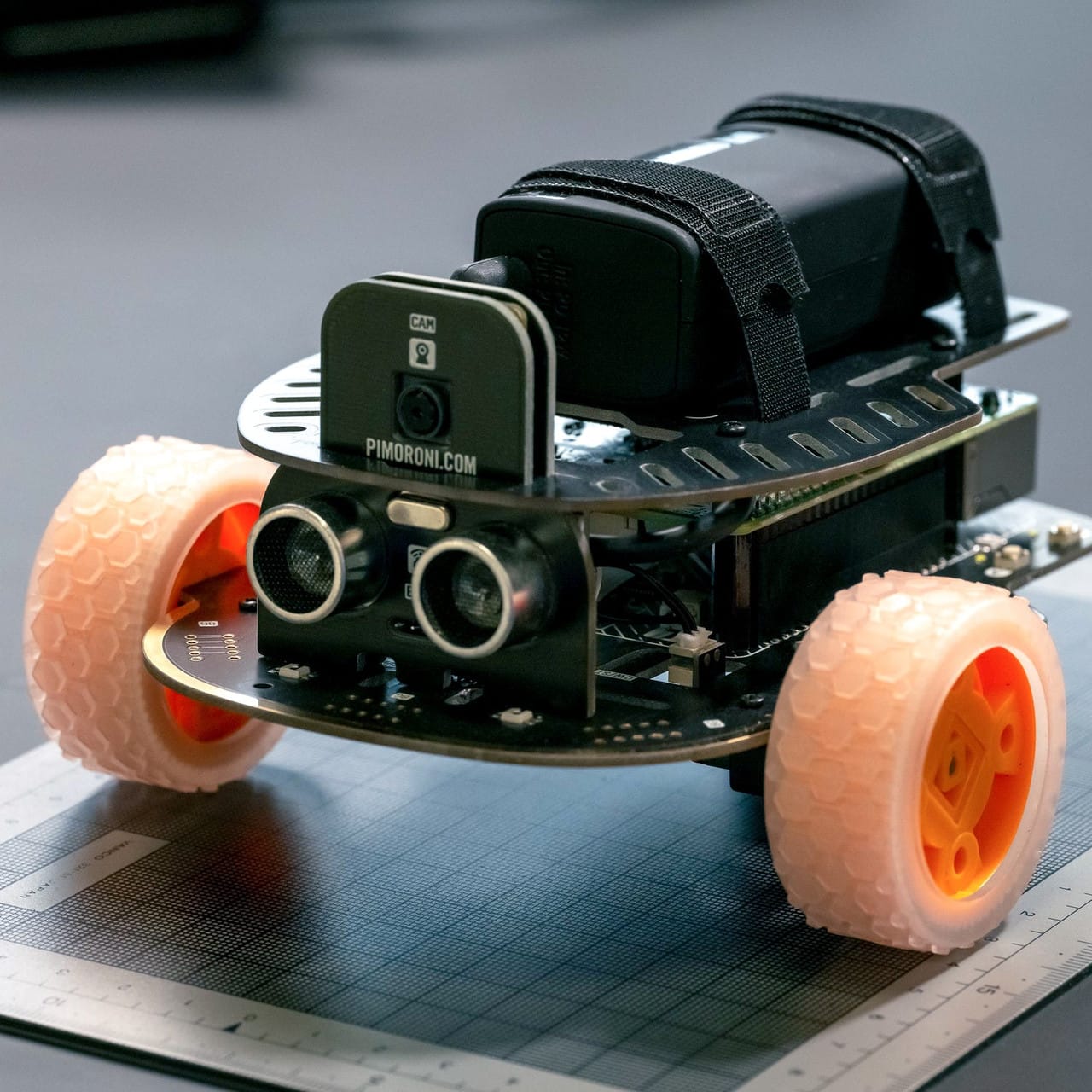
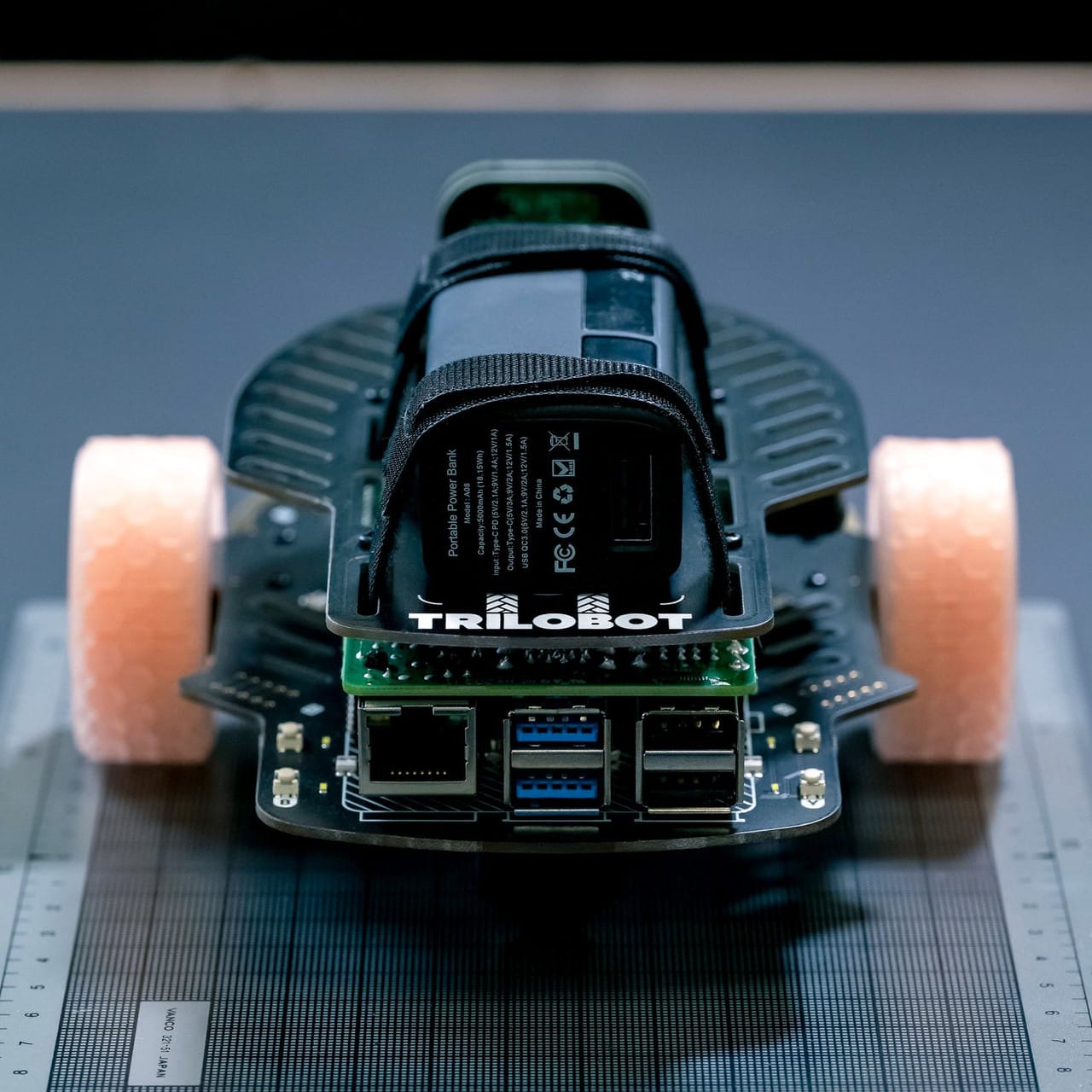
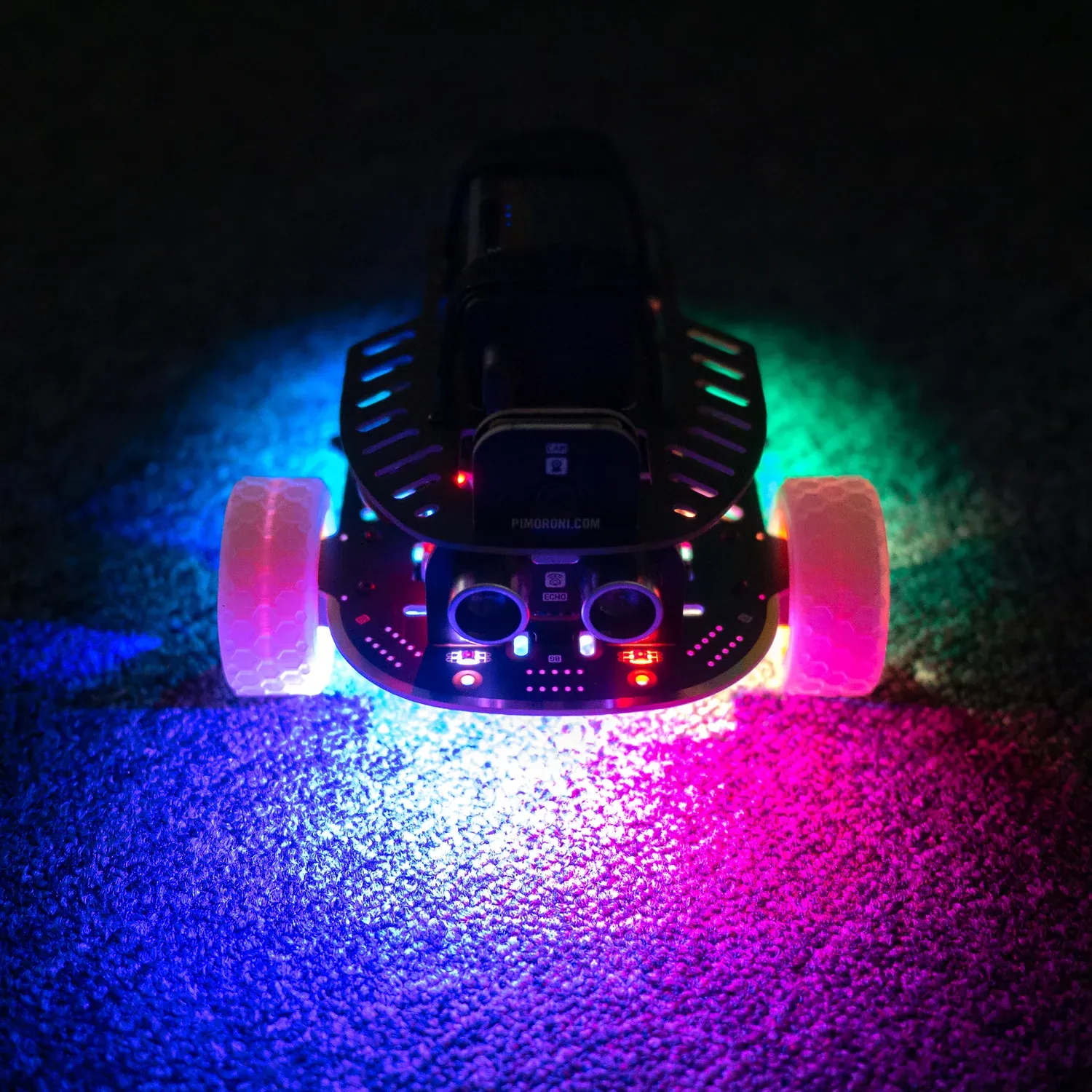
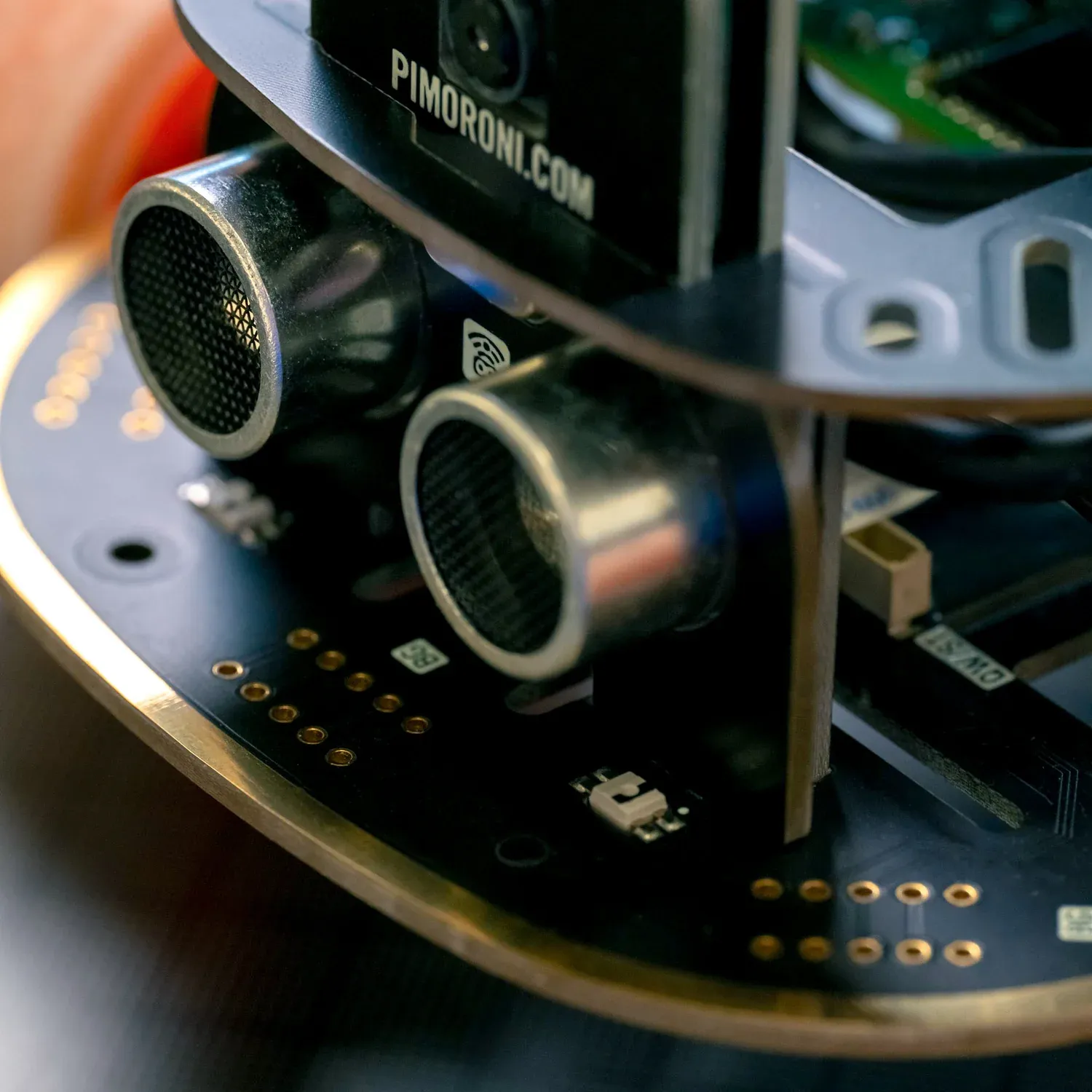
Pictures of the Trilobot from Pimoroni.
The QNX Trilobot Library
Our goal with this one is to give you a ready-to-go library to drive the Trilobot (pun intended) right out of the box with QNX. Unlike the open-source Trilobot library for Linux, which is written in Python, the QNX library is written in C – this should allow you to better integrate the robot control with other high-performance activities like machine learning or data collection.
Here's an example of how easy it is to control the Trilobot:
// Initialize the bot
initialize_bot();
// Read a button state (0 = pressed due to pull-up)
if (read_button(Button_A) == 0) {
printf("Button pressed\n");
} else {
printf("Button not pressed\n");
}
// Initialize an LED and turn it on
initialize_led(Led_Y);
set_led(Led_Y, 1); // Turn on the LED
// Initialize the ultrasonic sensor and get a reading
initialize_ultrasonic();
float distance;
sleep(2);
if (read_ultrasonic(&distance) == 0) {
printf("Distance: %.2f cm\n", distance);
} else {
printf("Error reading distance\n");
}
// Initialize the motor driver
if (initialize_motors() != GPIO_SUCCESS) {
printf("ERROR: Motor initialization failed.\n");
return EXIT_FAILURE;
}
// Enable the motors & move the bot
motor_enable();
motor_move_forward();
sleep(2);
motor_move_backward();
sleep(2);
motor_turn_left();
sleep(2);
motor_turn_right();
sleep(2);
// Stop the motors
motor_stop();
You can pick up a Trilobot from Pimoroni, PiShop, and other sites.
The open-source library initially written by QNX, plus samples for every hardware component, is found at gitlab.com/qnx/projects/trilobot.
Individual Hardware Components
From our work on the Trilobot we've broken out each of the hardware components (plus more) into their own very basic samples. These samples show how to drive the sensors or outputs directly, or how to drive them using interfaces like I2C or SPI.
You can find all of the sample code in Gitlab at gitlab.com/qnx/projects/hardware-component-samples.
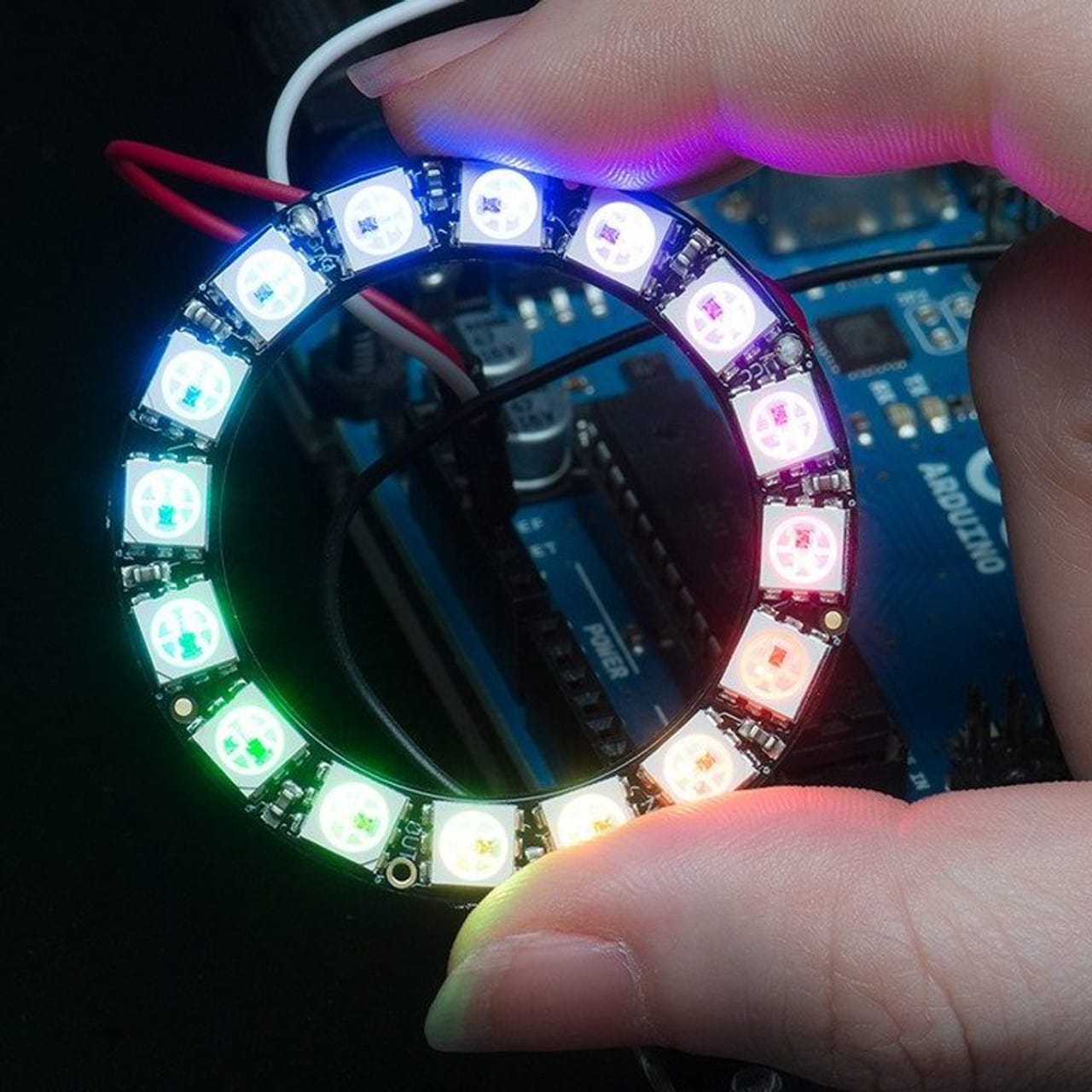
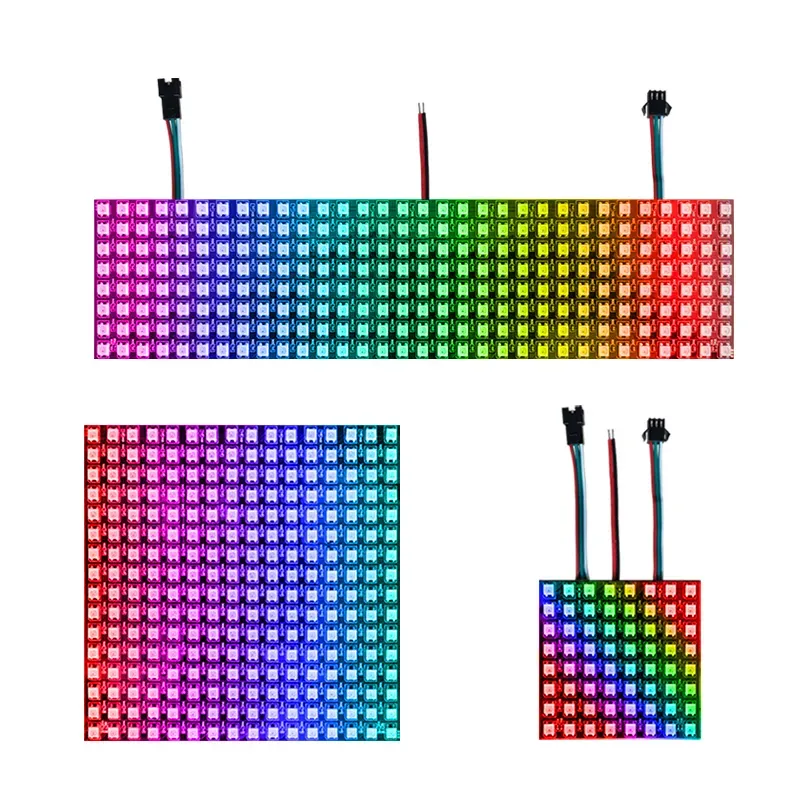
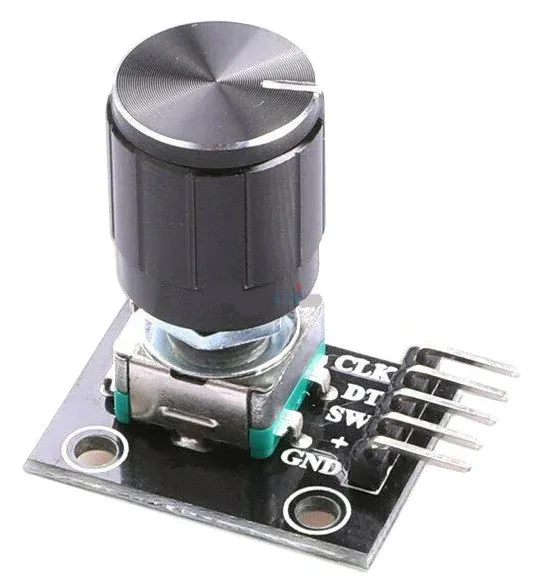
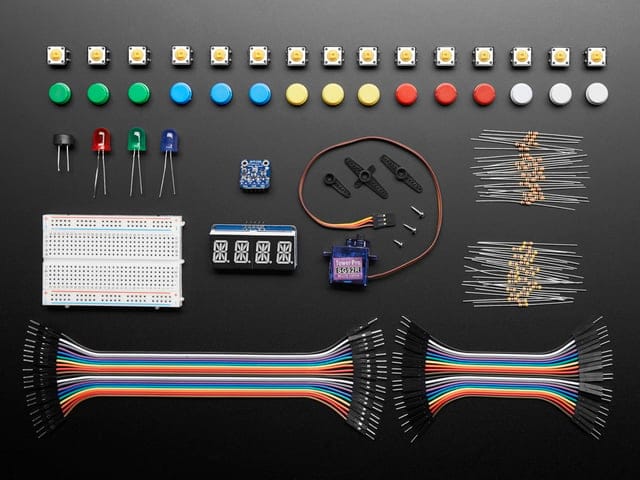
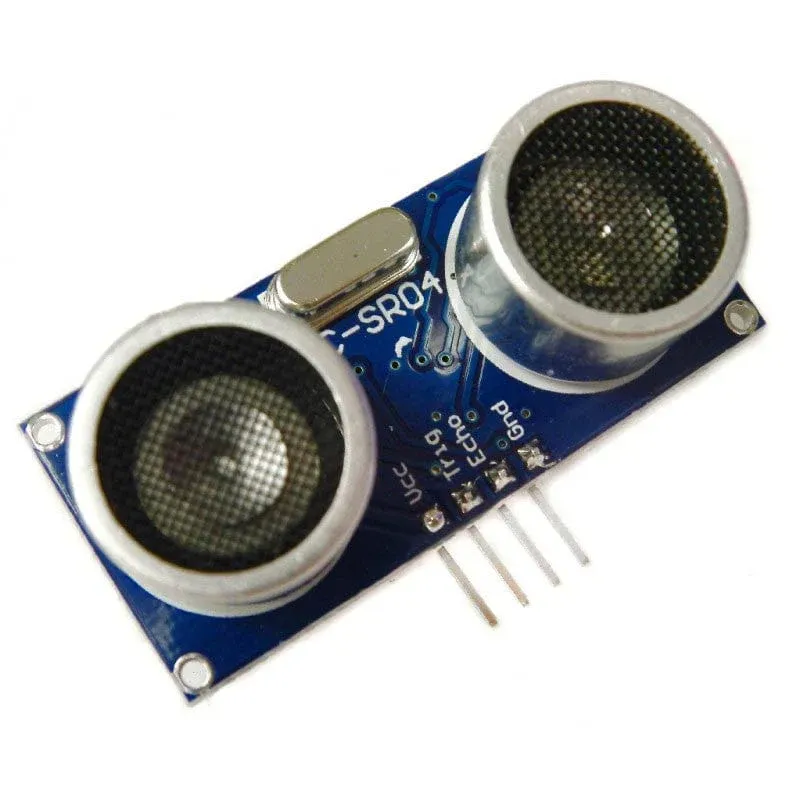
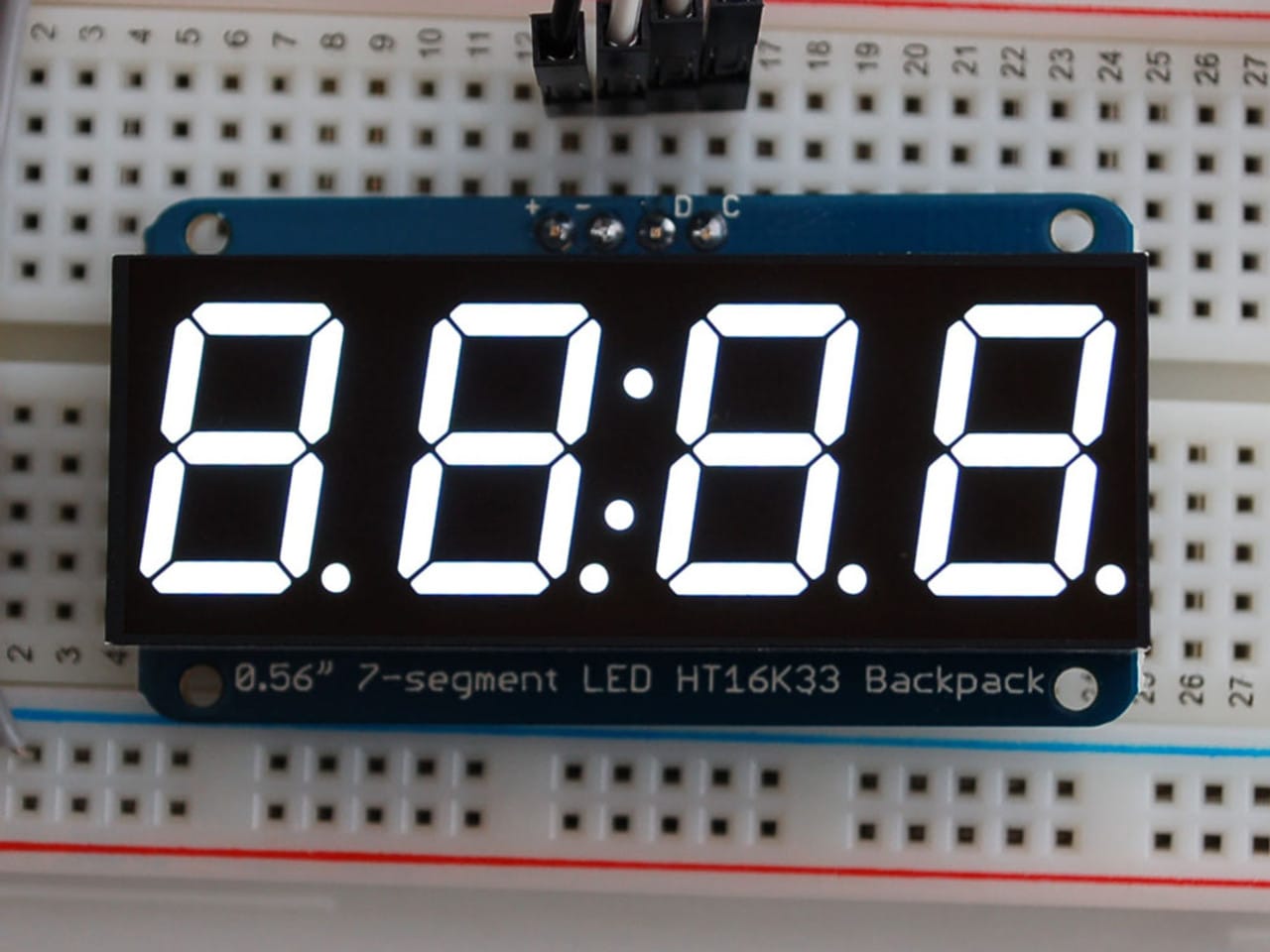
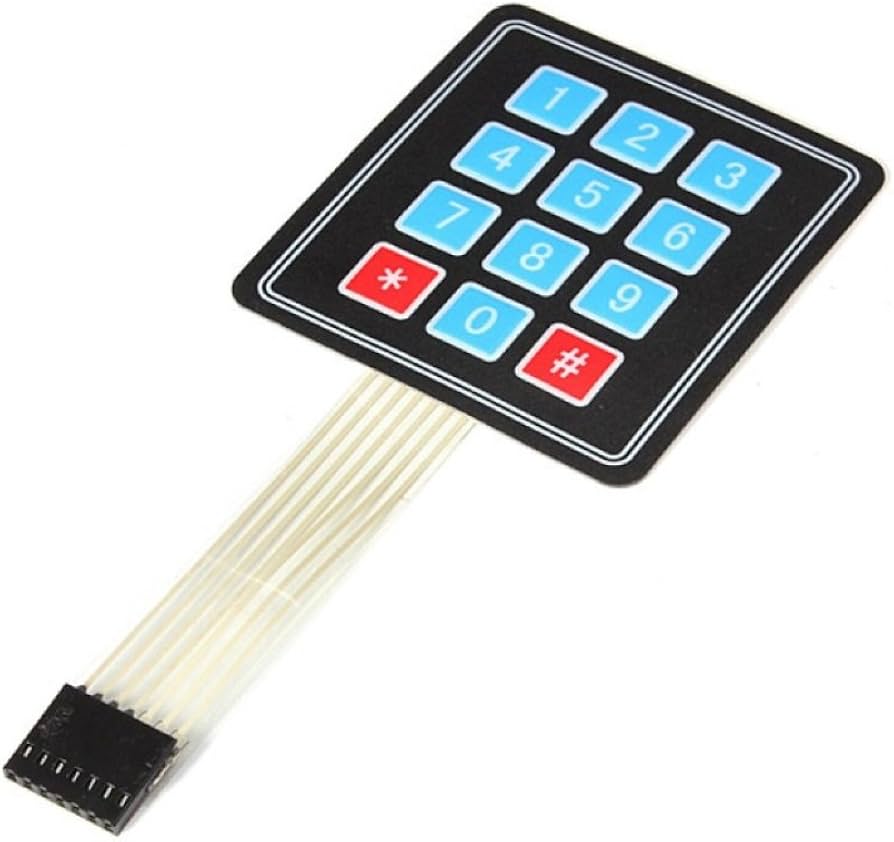
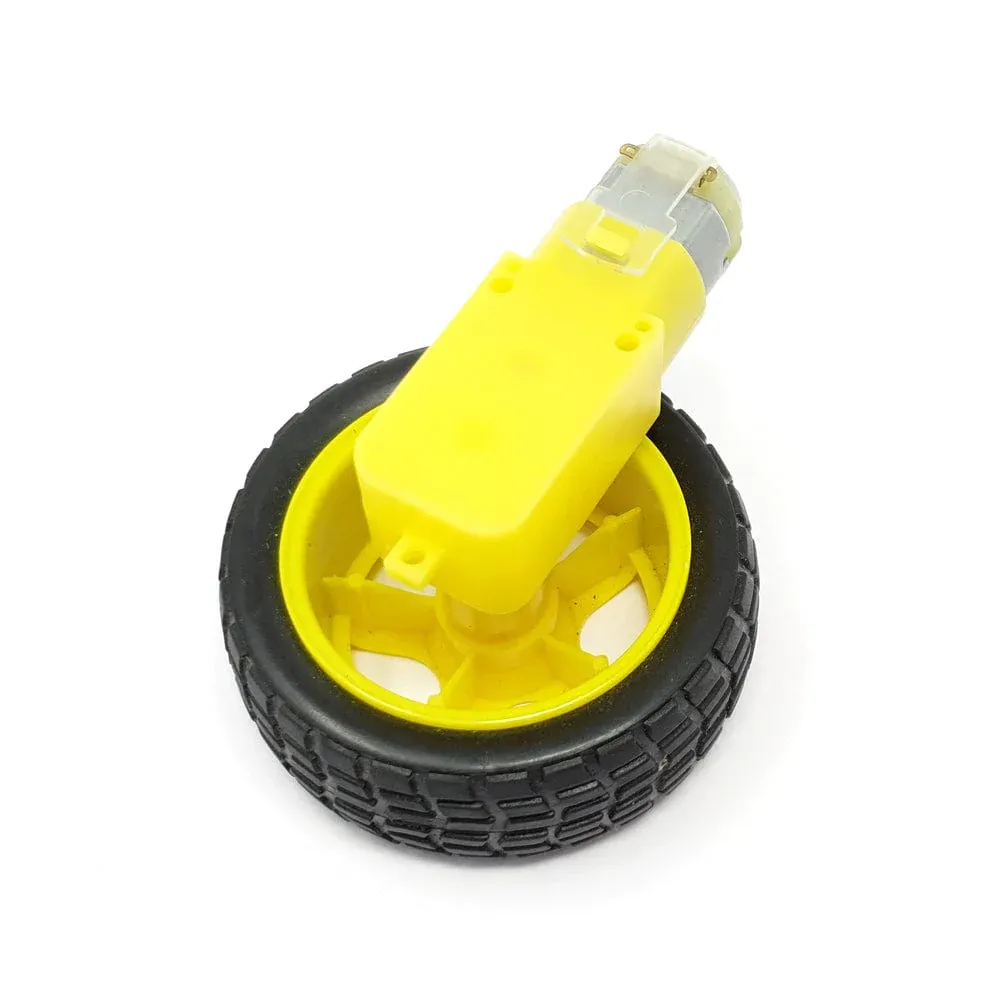
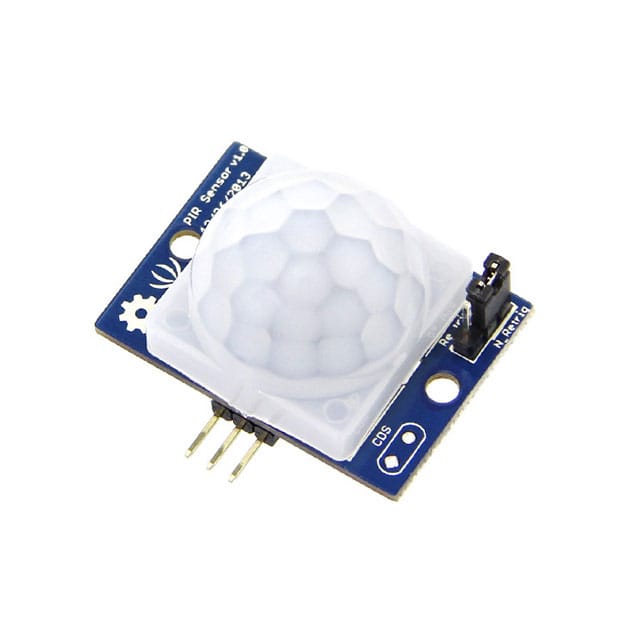
Some of the hardware components with QNX sample code.
Let's take a closer look at some of them.
LEDs – plain and RGB
There's several options in the samples to light up your projects:
- Regular LED – turn on or off a simple single LED
- 4-wire RGB LED – control color output to a 4-wire LED
- WS2812 RGB LED – drive small strips and large matrices of WS281x LEDs
- RGB LEDs on a SN3218A – like RGB LEDs on Trilobot
Inputs
Conversely to LEDs, here's some sample code for input options:
- Buttons – accept simple input from a button or switch
- Passive IR motion detector – trigger status changes when motion is detected
- Ultrasonic distance sensor – see how far away the object in front is
- Numeric keypad – like a security system, accept keypad input
Other outputs
- Drive motors! – drive motors using the DRV8833PWP
- Micro servos – move arms, spin indicators, etc, using servos and PWM
- Single 7-segment display – connect directly to a single-digit 7-segment display
- Use the single LED sample to trigger relays and more on/off outputs!
Coming Soon
The work doesn't end here – this repo will continue to evolve with added components and added functionality. Here's what we have on our short-term backlog:
- Rotary encoder – use a dial to process up/down input
- NFC card readers
- Multi-digit 7-segment displays over I2C
- Pimoroni PIM242 Rainbow HAT
- DHT22 temperature + humidity sensors
- OLED mini-displays over I2C (and maybe SPI)
- Additional robot educational platforms
- Camera
Make sure to watch the repos at gitlab.com/qnx/projects for more development!
Contributions
We absolutely welcome more contributions to these repos or to brand new repos in the Projects space. Just let us know what you're working on or feel free to fork and submit your own merge.